In this blog we are going to learn about how to show toast in lightning record page by using force:showToast. Toast are the message box or you can say notification which developer can show according to action of the user. Let’s know the attribute of toast.
ATTRIBUTES
- type : The toast can be of 4 types
- info
- success
- warning
- error
- key : It specify the icon on the toast when type is not defined.
- title : it specify the title of the toast
- message : It specifies the message to show the user
- messageTemplate : If we want to override the message which is specified in message attribute we can use this attribute along with messageTemplateData.
- messageTemplateData : To show the overridden message you must use this attribute.
- duration : It specify the duration of toast to be show to user. After specified duration toast will automatically disappear.
- mode : It specify how the toast will disappear. In salesforce there are 3 types of mode.
- dismissible : Toast will disappear either by user action or on complete of specified duration.
- pester : Toast will disappear on complete of specified duration. Close button will not appear.
- sticky : Toast will disappear by user action .
TOAST EXAMPLE
Create lightning component as follows:
<aura:component implements="flexipage:availableForRecordHome,force:hasRecordId" access="global" >
<div>
<lightning:button label="Information"
variant="brand"
onclick="{!c.showInfoToast}"
>
</lightning:button>
<lightning:button label="Error"
variant="destructive"
onclick="{!c.showErrorToast}">
</lightning:button>
<lightning:button label="Warning"
variant="inverse"
onclick="{!c.showWarningToast}">
</lightning:button>
<lightning:button label="Success"
variant="inverse"
onclick="{!c.showSuccessToast}">
</lightning:button>
</div>
</aura:component>
JavaScript Controller :
({
showInfoToast : function(component, event, helper) {
var toastEvent = $A.get("e.force:showToast");
toastEvent.setParams({
title : 'Info Message',
message: 'Mode is dismissible ,duration is 5sec and this is normal Message',
messageTemplate: 'Record {0} created! See it {1}!',
duration:' 5000',
key: 'info_alt',
type: 'info',
mode: 'dismissible'
});
toastEvent.fire();
},
showSuccessToast : function(component, event, helper) {
var toastEvent = $A.get("e.force:showToast");
toastEvent.setParams({
title : 'Success Message',
message: 'Mode is pester ,duration is 5sec and this is normal Message',
messageTemplate: 'Record {0} created! See it {1}!',
duration:' 5000',
key: 'info_alt',
type: 'success',
mode: 'pester'
});
toastEvent.fire();
},
showErrorToast : function(component, event, helper) {
var toastEvent = $A.get("e.force:showToast");
toastEvent.setParams({
title : 'Error Message',
message:'Mode is pester ,duration is 5sec and Message is not overrriden because messageTemplateData is not specified',
messageTemplate: 'Mode is pester ,duration is 5sec and Message is overrriden',
duration:' 5000',
key: 'info_alt',
type: 'error',
mode: 'pester'
});
toastEvent.fire();
},
showWarningToast : function(component, event, helper) {
var toastEvent = $A.get("e.force:showToast");
toastEvent.setParams({
title : 'Warning',
message: 'Mode is pester ,duration is 5sec and normal message',
messageTemplate: 'Mode is sticky ,duration is 5sec and Message is overrriden because messageTemplateData is {1}',
messageTemplateData: ['Salesforce', {
url: 'https://nikforce.blogspot.com/',
label: 'Click Here',
}],
duration:' 5000',
key: 'info_alt',
type: 'warning',
mode: 'sticky'
});
toastEvent.fire();
},
})
Output :
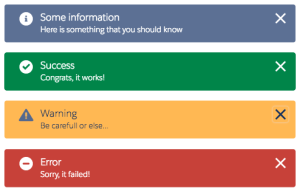